Optimizing MCX Gates - Preparing for Future Hardware Today
This note describes how to use the Classiq platform to create MCX gates, including one with 14 controls - a problem from our recent Coding Competition. Then, it demonstrates a much more complex example with 50 control qubits.
Quantum Resources are Valuable, Yet Limited
Quantum computers offer tantalizing promises to those who can harness their power. And though today’s computers are not quite able to solve real-world problems, those who are able to optimize for the hardware available will be able to reap rewards sooner than those who wait. The MCX gate is an important quantum gate used in a variety of circuits, such as the Grover Operator, logical AND operator, various state preparation algorithms, and arithmetic comparators. The ability to adapt implementations of MCX gates to meet the hardware constraints - limited qubit count, fidelities, gate count, and so on - is not trivial.
Take the example of an MCX gate with 4 control qubits. Two equivalent intermediate steps to decomposing this gate are shown below. While they achieve the same functionality, circuit 1 has a depth of 5, and circuit 2 has a depth of 4, as the first two gates can be performed in parallel - q0, q1, and q5 being operated on at the same time as q2, q3, and q6. While this example may seem irrelevant, with few problems miraculously solved when requiring one less operation, the scalability of this problem is extremely relevant. That one extra operation sums fast when handling massive MCX gates - the ones needed for real-world applications.

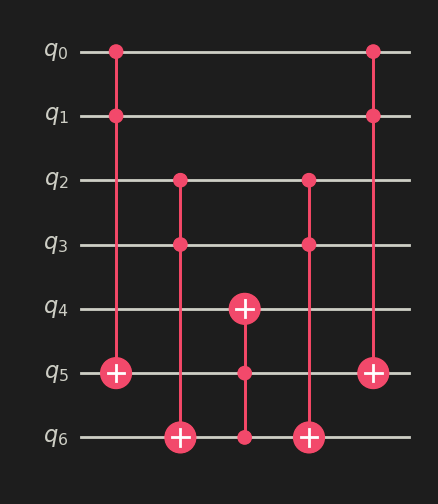
The 14-Control MCX Gate
In our recent coding competition, competitors were tasked with decomposing a 14-control MCX gate into single-qubit unitary gates and CX gates, while constrained to 20 total qubits, with all ancilla qubits released/uncomputed at the end. The winners were chosen based on the circuit with the shortest depth, as the potential for real-world problem solving is limited on this metric: with more noise introduced with each operation, the greater potential for inaccurate results.
How to Create a 14-Control MCX Gate with Classiq
Classiq offers two powerful options to creating optimized, hardware-aware circuits - a Python SDK and an extension in Visual Studio Code - with equivalent circuit functionalities. To create an MCX gate with 14 control qubits using Classiq’s Python SDK, we simply call the MCX function and declare the control qubit count. Then, to constrain this circuit to only 20 qubits and optimize for circuit depth, we declare our max width and optimization parameter within our ModelDesigner object.
Using Classiq’s Visual Studio Code extension, we declare our global constraints: use no more than 20 qubits and optimize for depth. We then call our MCX function with 14 control qubits, and we’re ready to synthesize.
The resulting interactive circuit, seen below and available here, was automatically generated in seconds and has a circuit depth of 95.
To see other solutions created by the competitors over the month-long competition, see here.
Beyond 14 Controls
The power of the Classiq synthesis engine is far greater than creating optimized, 14-control MCX gates in an instant. Take the following code, where we create an optimized MCX gate with 50 control qubits.
The 50-control MCX gate interactive circuit shown below can be found here.
Classiq's robust set of built-in functions includes far more than MCX gates, with each just as simple for the user to define, leaving the intense calculations and optimization techniques to Classiq’s synthesis engine. With Classiq, optimization happens beyond just the gate-level, with an awareness of high-level functional blocks and their interactions leading to revolutionary circuit design in moments.
Schedule a live demonstration of the Classiq platform to see it in action, or contact us to learn how you can create industry-leading quantum circuits in minutes.
This note describes how to use the Classiq platform to create MCX gates, including one with 14 controls - a problem from our recent Coding Competition. Then, it demonstrates a much more complex example with 50 control qubits.
Quantum Resources are Valuable, Yet Limited
Quantum computers offer tantalizing promises to those who can harness their power. And though today’s computers are not quite able to solve real-world problems, those who are able to optimize for the hardware available will be able to reap rewards sooner than those who wait. The MCX gate is an important quantum gate used in a variety of circuits, such as the Grover Operator, logical AND operator, various state preparation algorithms, and arithmetic comparators. The ability to adapt implementations of MCX gates to meet the hardware constraints - limited qubit count, fidelities, gate count, and so on - is not trivial.
Take the example of an MCX gate with 4 control qubits. Two equivalent intermediate steps to decomposing this gate are shown below. While they achieve the same functionality, circuit 1 has a depth of 5, and circuit 2 has a depth of 4, as the first two gates can be performed in parallel - q0, q1, and q5 being operated on at the same time as q2, q3, and q6. While this example may seem irrelevant, with few problems miraculously solved when requiring one less operation, the scalability of this problem is extremely relevant. That one extra operation sums fast when handling massive MCX gates - the ones needed for real-world applications.

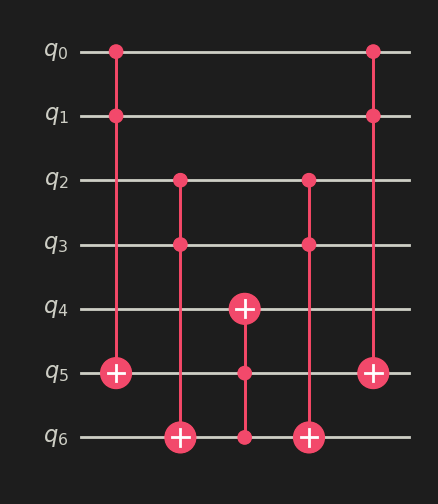
The 14-Control MCX Gate
In our recent coding competition, competitors were tasked with decomposing a 14-control MCX gate into single-qubit unitary gates and CX gates, while constrained to 20 total qubits, with all ancilla qubits released/uncomputed at the end. The winners were chosen based on the circuit with the shortest depth, as the potential for real-world problem solving is limited on this metric: with more noise introduced with each operation, the greater potential for inaccurate results.
How to Create a 14-Control MCX Gate with Classiq
Classiq offers two powerful options to creating optimized, hardware-aware circuits - a Python SDK and an extension in Visual Studio Code - with equivalent circuit functionalities. To create an MCX gate with 14 control qubits using Classiq’s Python SDK, we simply call the MCX function and declare the control qubit count. Then, to constrain this circuit to only 20 qubits and optimize for circuit depth, we declare our max width and optimization parameter within our ModelDesigner object.
Using Classiq’s Visual Studio Code extension, we declare our global constraints: use no more than 20 qubits and optimize for depth. We then call our MCX function with 14 control qubits, and we’re ready to synthesize.
The resulting interactive circuit, seen below and available here, was automatically generated in seconds and has a circuit depth of 95.
To see other solutions created by the competitors over the month-long competition, see here.
Beyond 14 Controls
The power of the Classiq synthesis engine is far greater than creating optimized, 14-control MCX gates in an instant. Take the following code, where we create an optimized MCX gate with 50 control qubits.
The 50-control MCX gate interactive circuit shown below can be found here.
Classiq's robust set of built-in functions includes far more than MCX gates, with each just as simple for the user to define, leaving the intense calculations and optimization techniques to Classiq’s synthesis engine. With Classiq, optimization happens beyond just the gate-level, with an awareness of high-level functional blocks and their interactions leading to revolutionary circuit design in moments.
Schedule a live demonstration of the Classiq platform to see it in action, or contact us to learn how you can create industry-leading quantum circuits in minutes.
About "The Qubit Guy's Podcast"
Hosted by The Qubit Guy (Yuval Boger, our Chief Marketing Officer), the podcast hosts thought leaders in quantum computing to discuss business and technical questions that impact the quantum computing ecosystem. Our guests provide interesting insights about quantum computer software and algorithm, quantum computer hardware, key applications for quantum computing, market studies of the quantum industry and more.
If you would like to suggest a guest for the podcast, please contact us.